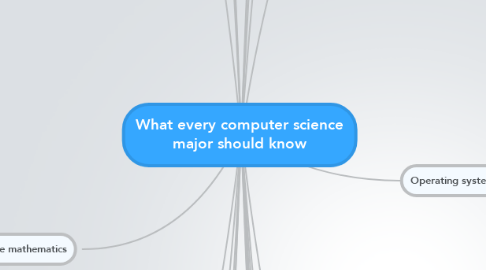
1. http://matt.might.net/articles/what-cs-majors-should-know/
2. build a portfolio
2.1. publicly browsable code
2.2. per-project page
2.3. github or Google code
3. Systems administration
3.1. Install and administer a Linux distribution
3.2. Configure and compile the Linux kernel
3.3. Troubleshoot a connection with dig, ping and traceroute
3.4. Compile and configure a web server like apache
3.5. Compile and configure a DNS daemon like bind
3.6. Maintain a web site with a text editor
3.7. Cut and crimp a network cable
4. Programming languages
4.1. best way to learn how to learn progamming languages is to learn multiple programming languages and programming paradigms
4.2. difficulty of learning the nth language is half the difficulty of the (n-1)th
4.3. every computer science major should implement an interpreter
4.4. Ideally, every computer science major would take a compilers class
4.5. specific languages
4.5.1. Racket (dialect of Lisp)
4.5.2. C
4.5.3. Javascript
4.5.4. Squeak
4.5.5. Java
4.5.6. Satandard ML
4.5.7. Prolog
4.5.8. Scala
4.5.9. Haskell
4.5.10. C++
4.5.11. Assembly
4.6. recommendations
4.6.1. generative programming (macros)
4.6.2. lexical (and dynamic) scope
4.6.3. closures
4.6.4. continuations
4.6.5. higher-order functions
4.6.6. dynamic dispatch
4.6.7. subtyping
4.6.8. modules and functors
4.6.9. monads as semantic concepts distinct from any specific syntax
5. Discrete mathematics
5.1. formal mathematical notation
5.2. sets
5.3. tuples
5.4. sequences
5.5. functions
5.6. power sets
5.7. trees
5.8. graphs
5.9. formal languages
5.10. automata
5.11. learn enough number theory to study and implement common cryptographic protocols
6. Data structures and algorithms
6.1. how to design algorithms
6.1.1. greedy
6.1.2. dynamic strategies
6.2. know both the imperative and functional versions of each algorithm
6.3. hash tables
6.4. linked lists
6.5. trees
6.6. binary search trees
6.7. directed and undirected graphs
7. Security
7.1. social engineering
7.2. buffer overflows
7.3. integer overflow
7.4. code injection vulnerabilities
7.5. race conditions
7.6. privilige confusion
8. Cryptography
8.1. Understand
8.1.1. symmetric-key cryptosystems
8.1.2. public-key cryptosystems
8.1.3. secure hash functions
8.1.4. challenge-response authentication
8.1.5. digital signature algorithms
8.1.6. threshold cryptosystems
8.2. recommendations
8.2.1. breaking ciphertext using pre-modern cryptosystems with hand-rolled statistical tools
8.2.2. implemment RSA
8.2.3. create their own digital certificate and set up https in apache
8.2.4. write a console web client that connects over SSL
8.2.5. know how to use GPG
8.2.6. know how to use public-key authentication for ssh
8.2.7. know how to encrypt a directory or a hard disk
9. User experience design
10. Visualization
11. Technical communication
12. An engineering core
12.1. physics through electromagnetism
12.2. take up through multivariate calculus
12.3. differential equations
12.4. probability
12.5. linear algebra
12.6. statistics
13. The Unix philosophy
13.1. emphasizes linguistic abstraction and composition in order to effect computation
13.2. command-line computing
13.3. IDE-less software development
13.4. text-file configuration
13.5. recommendations
13.5.1. navigate and manipulate the filesystem
13.5.2. compose processes with pipes
13.5.3. comfortably edit a file with emacs and vim
13.5.4. create, modify and execute a Makefile for a software project
13.5.5. write simple shell scripts
14. Theory
14.1. Computational complexity
14.1.1. understand the difference between P, NP, NP-Hard and NP-Complete
14.2. models of computation
14.2.1. finite-state automata
14.2.2. regular languages (and regular expressions)
14.2.3. pushdown automata
14.2.4. context-free languages
14.2.5. formal grammars
14.2.6. Turing machines
14.2.7. lambda calculus
14.2.8. undecidability
15. Architecture
15.1. understand a computer from the transistors up
15.2. standard levels of abstraction
15.2.1. transistors
15.2.2. gates
15.2.3. adders
15.2.4. adders
15.2.5. flip flops
15.2.6. ALUs
15.2.7. control units
15.2.8. caches
15.2.9. RAM
15.3. GPU model of high-performance computing
15.4. recommendations
15.4.1. design and simulate a small CPU
16. Operating systems
16.1. recommendations
16.1.1. print "hello world" during the boot process
16.1.2. design their own scheduler
16.1.3. modify the page-handling policy
16.1.4. create their own filesystem
16.2. kernel
16.2.1. system calls
16.2.2. paging
16.2.3. scheduling
16.2.4. context-switching
16.2.5. filesystems
16.2.6. internal resource management
17. Networking
17.1. network stack and routing protocols within a network
17.2. mechanics of building an efficient, reliable transmission protocol (like TCP) on top of an unreliable transmission protocol (like IP)
17.3. understand the trade-offs involved in protocol design--for example, when to choose TCP and when to choose UDP
17.4. understand exponential back off in packet collision resolution and the additive-increase multiplicative-decrease mechanism involved in congestion control
17.5. recommendations
17.5.1. protocols
17.5.1.1. 802.3 and 802.11
17.5.1.2. IPv4 and IPv6
17.5.1.3. DNS, SMTP and HTTP
17.5.2. implement
17.5.2.1. an HTTP client and daemon
17.5.2.2. a DNS resolver and server
17.5.2.3. a command-line SMTP mailer
17.5.3. network sniffing w wireshark
18. Software testing
19. Parallelism
19.1. architecture
19.1.1. multicore
19.1.2. caches
19.1.3. buses
19.1.4. GPUs